Python is undoubtedly one of the most popular programming languages in today’s world. With its quickly updated libraries and the ease to code, Python has managed to make its place in the rapidly growing technology era.
You can refer to the Python handbook that has everything from basic learning to gaining knowledge about Pandas along with a lot of direct examples. With this book, you can learn the most relevant information before starting to practically use the Python language. This book is also meant for those programmers who want to quickly refresh their knowledge on Python for data analysis. One of the best parts is that it is available for FREE.
Let us find out some commonly asked questions on Python programming:
- What is Python programming?
- Benefits of Python in trading
- Applications of Python in trading
- Running of Python scripts
- What is syntax?
- What is indentation?
- What are variables and operators?
- Conditional statements and loops
- What are functions?
- Modules, packages and libraries
What is Python programming?
Python is a programming language that places weight on coding productivity and code readability. Python makes use of coding which looks like written English. Moreover, the coding is done in words and sentences, rather than characters.
Free self-paced course
An example of a simple Python code goes as follows:
Output:
5
In trading, Python provides several benefits. Let us find out what are those points that make Python popular.
Benefits of Python in trading
Python is known to be a preferred language for developing trading strategies by programmers/developers since it provides benefits such as:
- Python has certain APIs and libraries for machine learning as well as data science that make the analysis smoother as compared to other languages.
- It helps the trader with quick and easy coding for importing data and for data visualization in the form of graphs.
- Most quant traders prefer Python as it helps them build their own data connectors, execution mechanisms, backtesting, risk and order management, walk forward analysis and optimization testing modules.
- First updates to Python trading libraries are a regular occurrence in the developer community.
Next, let us find out about applications of Python in trading.
Applications of Python in trading
Python, like any other programming language, can help an algorithmic trader to trade in the stock market with minimum human intervention. Python helps you program the trading system with the Python codes (set of instructions) for executing the trade orders. With the help of different codes, you can program the system to do the data analysis, find out the best entry and exit positions and then execute the trade.
Hence, apart from Python’s extensive use in other fields like medicine, marketing etc. it is used for algorithmic trading across the globe.
Technically speaking, Python has a huge significance in the overall trading process as python codes are used to develop quantitative models for trading strategies.
Python trading has gained traction in the quant finance community as it makes it easy to build intricate statistical models due to the availability of sufficient scientific libraries like Pandas, NumPy, scikit-learn and more.
Take a look at the areas where Python is applicable during algorithmic trading:
Quantitative data analysis
For executing the trade order, the first step consists of acquiring the data for the particular stock and then conducting a quantitative data analysis. Quantitative analysis is the process that helps to find out the performance of the strategy in the market by using statistical tools such as machine learning, neural networks, etc. In order to develop your skills, understand the various applications of neural network in trading with a well curated course.
Developing trading algorithms
Developing trading algorithms implies converting trading hypotheses into trading algorithms based on specific rules of entry/exit. These algorithms are developed with the help of coding in Python.
Automate your trades using Python
Free self-paced course
Backtesting
It has never been easier to backtest your trading strategies as much as it is with Python. With just a few lines of code, Python backtests your trading strategies on historical data. You can implement your trading strategies live after converting them to an event-driven strategy type using a platform like Blueshift.
Evaluating trading strategies
Next step is to evaluate the trading strategies after the backtest. Now Python coding aids by helping to find out how beneficial the strategy will be after being deployed. For evaluating the trading strategy we can calculate metrics such as annualised return, annualised volatility, sharpe ratio etc. On the basis of these figures, you can measure the possible return and risks of deploying the trading strategy. We have covered the explanation of each in detail in our blog article on Python for Trading: An Introduction.
Further, Python also helps with integration of the strategy code with broker API for taking the strategy live.
Going forward, let us take a look at how the Python scripts are run.
Running of Python scripts
For running the scripts in Python, a Python environment is needed. An environment consists of an interpreter for Python standard libraries and pre-installed packages. Anaconda is this environment where Jupyter is the framework using which you can code in Python and run your scripts for the desirable output. Also, Spyder is an IDE or Integrated development environment which is used for running python codes as well.
For installing Anaconda and setting up a Python environment, you can refer to the blog on setting up Python.
Let us take a look at the example below:
How would you make Python print "Hello World" for you? Well, it's never been this easy, just use the print command:
Output:
Hello World!
Output:
I am new to programming!
Python is cool!
Running the Python scripts is simple and fun! Since you do not need an extremely strong computer science background for Python programming, it is a favoured language for almost all beginners.
Going forward, let us find out about syntax in the next section.
Start your Python Programming journey with this FREE course
What is syntax?
Syntax implies the set of rules which define how the program will be written and interpreted.
For example, this is the Python syntax:
print (“Welcome, John!”)
In the above example, syntax implies the characters such as opening and closing brackets () and the inverted commas “” as well as the keyword “print”.
Output:
Welcome, John!
Next, let us find out what indentation is.
What is indentation?
Python uses indentation to identify the different blocks of code. The white space indicates the indentation. All the codes with the same spaces on the left belong to the same block of code. Usually, the number of spaces on the left are four.
Take a look at the example below:

Output:
64
In the example above, since the second line of code is a separate expression, the indentation is not correct. Hence, when you run the code it throws an error. But when you remove the space/indentation, the correct output is shown.
Next, we will learn about the basic coding requirements which are variables and operators.
Free self-paced course
What are variables and operators?
Variables
Variables are characters or words that are given a user-defined value. Variables, as the name suggests can be varied during the execution of the code. They are allocated a space in the computer memory based on their data type. Python doesn't require explicit declaration of the data type for a variable.
Example 1
Output:
45
1456.8
Alex
Example 2
Output:
100
Changing the value of a variable
Output:
Original value: 100
Updated value: 120.3
Operators
Operators are used on the values and variables in Python for performing certain operations.
Take a look at different types of operators:
- Arithmetic operators
- Relational operators
- Logical operators
- Operator precedence
Arithmetic operators
Arithmetic operators are used to perform mathematical operations like addition, subtraction, multiplication, division, modulus, exponentiation and floor division.
Automate your trades using Python
Free self-paced course
For example:
Output:
12
4
32
Relational operators
Relational operators compare the values of two expressions and return true or false.
For example:
Output:
Is a greater than b?: False
Is a smaller than b?: True
Is a equal to b?: False
Is a not equal to b?: True
Is a greater than or equal to b?: False
Is a smaller than or equal to b?: True
Logical operators
Logical operators perform Logical AND, Logical OR and Logical NOT operations.
For example:
If A, B and the combinations are as follows:
Case |
A |
B |
A and B print(A and B) |
A or B print(A or B) |
Not A print(Not A) |
Not B print(Not B) |
1 |
True |
False |
False |
True |
False |
True |
2 |
True |
True |
True |
True |
False |
False |
3 |
False |
True |
False |
True |
True |
False |
4 |
False |
False |
False |
False |
True |
True |
In the table above, you can see how AND, OR and NOT are giving output.
Operator precedence
This is used in an expression with more than one operator to find out as to which operation to perform first.
Start your Python Programming journey with this FREE course
Take a look at the table below to find out all the operators of different precedence:
Operator in characters |
Name of the operator |
() |
Parentheses |
** |
Exponent |
* , /, % |
Multiplication, division, modulus |
+- |
Addition, subtraction |
<<= >>= |
Relational less than/less than or equal to Relational greater than/greater than or equal to |
is, is not in, in not |
Identity Membership operators |
not |
Logical NOT |
and |
Logical AND |
or |
Logical OR |
For example:
Output:
Hello! Welcome.
Next comes the section explaining conditional statements and loops.
Conditional statements and Loops
Conditional statements in Python are mainly handled by IF and else statements. They help to perform different operations on the basis of the nature of the output. Basically, the output is decided by evaluating True or False for the condition followed by the “if” keyword.
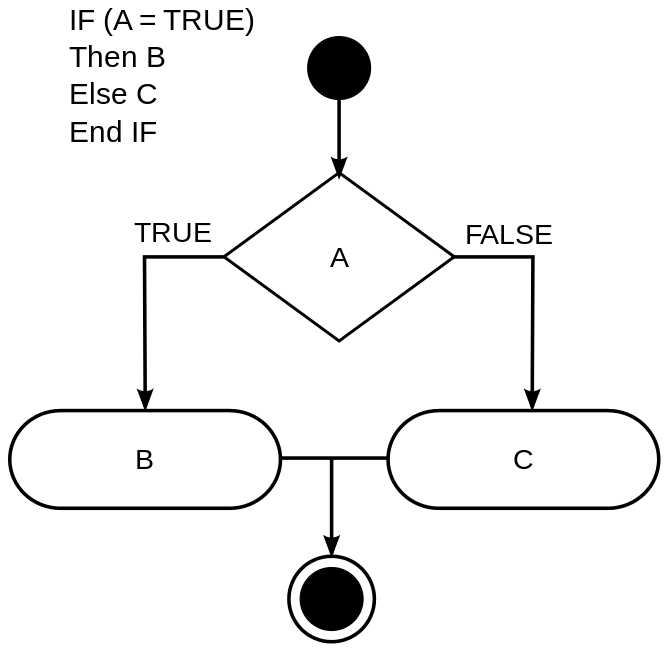
In Python, the syntax for an ‘if else' conditional statement is as follows:
if (condition_1):
print("statement_block_1")
else:
print("statement_block_2")
Take a look at the example below:
Output:
We will sell the stock and book the profit
If you change the value of the variable 'stock_price_ABC' to...
Output:
We will keep buying the stock
Automate your trades using Python
Free self-paced course
Loops are a part of conditional statements. Loops execute a set of statements repeatedly till the given condition is satisfied in the code. Once the condition becomes false, we exit the loop and move to the next block of code.
For example:
Output:
1
2
3
Moving to the next section let us find out about functions.
What are functions?
A function is a reusable block of code that does the same thing each time it is executed (or technically speaking, "called"). A function can take multiple inputs, called parameters, or no inputs at all. It can also be used to return a certain value.
The syntax for constructing a function is:
def function_name (parameter-list):
Statements, i.e function body
return a value, if required
For example:
Since code above has already defined the function, you can insert the values for x and n for getting x raised to n in the output every time by calling the function.
Let us see how:
Output:
1000
125
Next, let us find out about modules, packages and libraries in Python.
Modules, Packages and Libraries
Modules
A module is a file consisting of Python definitions and statements. A module basically defines functions, classes and variables. Also, a module can include a code that can be run in the Python environment. Modules help to keep the codes organized in one place.
Why do we need modules?
As a code gets longer, we may want to split it into several small files for easier maintenance. Also, we may want to use a handy function that we have written in several programs without copying its definition into each program. To support this, Python has a way to put a code definition in a file and use them in another script or directly in an interactive instance of the interpreter. Such a file is called a module; definitions from a module can be imported into other modules or in the program that we code.
Free self-paced course
Packages
A package is basically a directory with Python files and a file with the name __init__ . py.
Hence, each directory inside of the Python path, which contains a file name __init__ . py, will be a package in Python.
Packages can be considered as a collection of modules. It is a way of structuring Python’s module namespace by using "dotted module names". For example, the module name matplotlib.pyplot designates a submodule named pyplot in a package named matplotlib.
As we know Python is an open-source project. The Python developers community make their codes available for others in the form of packages under the open source license.
Libraries
Libraries are a collection of reusable modules or functions which can be directly used in our code to perform a certain task without the necessity to write the code from scratch.
Python has a huge collection of libraries which can be used for various functionalities like computing, machine learning, data visualization, etc. However, we will talk about the most relevant libraries required for coding trading strategies.
We will be required to:
- Import financial data,
- Perform numerical analysis,
- Build trading strategies,
- Plot graphs, and
- Perform backtesting on data
For all these functions, here are a few most widely used libraries:
- NumPy – NumPy or NumericalPy, is mostly used to perform numerical computing on arrays of data. The array is an element which contains a group of elements and we can perform different operations on it using the functions of NumPy.
- Pandas – Pandas is mostly used with DataFrame, which is a tabular or a spreadsheet format where data is stored in rows and columns. Pandas can be used to import data in various file formats to perform data analysis and manipulation of the tabular data.
- Matplotlib – Matplotlib is used to plot graphs like bar charts, scatter plots, histograms etc. It consists of various functions to modify the graph according to our requirements.
- TA-Lib – TA-Lib or Technical Analysis library is an open-source library and is extensively used to perform technical analysis on financial data using technical indicators such as RSI (Relative Strength Index), Bollinger bands, MACD etc.
You can learn more on Python programming with courses such as Python for trading:Basic and Python for Trading!. These courses provide you with a deeper insight into Python programming and its basics.
Take a look at this video to get a better understanding about the course on Python for trading:Basic -
Conclusion
Python is a simple programming language that can be used by anyone even without a background in programming. With knowledge and experience, one can use Python for quantitative analysis, algorithmic trading, backtesting etc.
In case you are also interested in developing lifelong skills that will always assist you in improving your trading strategies. In this algo trading course, you will be trained in statistics & econometrics, programming, machine learning and quantitative trading methods, so you are proficient in every skill necessary to excel in quantitative & algorithmic trading. Know more about the EPAT course now!
Downloadable file
Readers can download the Jupyter notebook with the Python codes mentioned in the blog with this downloadable button below.
Disclaimer: All data and information provided in this article are for informational purposes only. QuantInsti® makes no representations as to accuracy, completeness, currentness, suitability, or validity of any information in this article and will not be liable for any errors, omissions, or delays in this information or any losses, injuries, or damages arising from its display or use. All information is provided on an as-is basis.